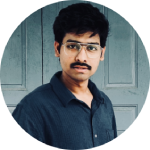
HTML Beginner Course
CSS Introduction
- CSS (Cascading Style Sheets): Used to style HTML elements.
- Purpose: Control layout, colors, fonts, and more.
- Syntax: Consists of selectors and declarations (property-value pairs).
Syntax:
selector {
property: value;
}
Code Example:
body {
background-color: lightblue;
}
Exercise:
- Create a CSS file to change the background color of an HTML page to light blue.
CSS Syntax
- Selectors: Target HTML elements (e.g., element selectors, class selectors, ID selectors).
- Properties: Define the style (e.g.,
color
,font-size
). - Values: Specify the style settings (e.g.,
red
,16px
).
Syntax:
selector {
property: value;
}
Code Example:
h1 {
color: red;
font-size: 24px;
}
Exercise:
- Style all
<h1>
elements to have red text and a font size of 24 pixels.
CSS Integration HTML Document
- Inline CSS: Use
style
attribute directly in HTML tags. - Internal CSS: Include CSS within the
<style>
tag in the HTML<head>
. - External CSS: Link to an external CSS file using
<link>
.
Syntax:
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<h1>This is a Heading</h1>
<p>This is a paragraph.</p>
</body>
</html>
Code Example:
/* styles.css */
h1 {
color: blue;
}
p {
color: green;
}
Exercise:
- Create an HTML file linked to an external CSS file. Style the
<h1>
and<p>
elements with different colors.
CSS Colors
- Color Values: Use names, hex codes, RGB, RGBA, HSL, HSLA.
- Color Keywords: Basic color names (e.g.,
red
,blue
). - Hex Codes: Represent colors as hexadecimal values (e.g.,
#ff0000
). - RGB/RGBA: Define colors using RGB values (e.g.,
rgb(255,0,0)
), with alpha for transparency (e.g.,rgba(255,0,0,0.5)
). - HSL/HSLA: Define colors using hue, saturation, lightness, and alpha (e.g.,
hsl(0, 100%, 50%)
,hsla(0, 100%, 50%, 0.5)
).
Syntax:
p {
color: #ff6347; /* Tomato */
}
h2 {
color: rgb(0, 255, 0); /* Lime */
}
div {
color: rgba(0, 0, 255, 0.5); /* Semi-transparent Blue */
}
span {
color: hsl(120, 100%, 50%); /* Green */
}
Exercise:
- Apply different color values to text elements on your webpage using color names, hex codes, RGB, and HSL.
CSS Backgrounds
- Background Color: Set the background color of elements
(
background-color
). - Background Image: Add images as backgrounds (
background-image
). - Background Repeat: Control image repetition (
background-repeat
). - Background Position: Position the background image
(
background-position
). - Background Size: Adjust the size of the background image
(
background-size
).
Syntax:
div {
background-color: lightgray;
background-image: url('background.jpg');
background-repeat: no-repeat;
background-position: center;
background-size: cover;
}
Exercise:
- Style a
div
with a background color and a background image. Adjust its repeat, position, and size properties.
CSS Borders
- Borders: Add borders to elements (
border
). - Properties:
border-width
: Thickness of the border.border-style
: Style of the border (e.g.,solid
,dashed
,dotted
).border-color
: Color of the border.
Syntax:
div {
border-width: 2px;
border-style: solid;
border-color: black;
}
Code Example:
p {
border: 1px solid red;
}
Exercise:
- Add a border to a
<div>
with different styles and colors, and experiment with border-width and border-color.
CSS Paddings
- Padding: Space between the element's content and its border
(
padding
). - Properties: Set padding for all sides or individual sides
(
padding-top
,padding-right
,padding-bottom
,padding-left
).
Syntax:
div {
padding: 20px; /* All sides */
padding-top: 10px; /* Top side only */
padding-right: 15px; /* Right side only */
}
Code Example:
section {
padding: 15px;
}
Exercise:
- Add padding to a
<section>
element and adjust the padding values for different sides.
CSS Margins
- Margins: Space outside the border, between elements (
margin
). - Properties: Set margin for all sides or individual sides (
margin-top
,margin-right
,margin-bottom
,margin-left
).
Syntax:
div {
margin: 30px; /* All sides */
margin-bottom: 20px; /* Bottom side only */
}
Code Example:
article {
margin: 20px;
}
Exercise:
- Apply margins to an
<article>
element and experiment with different margin values for each side.
CSS Outline
- Outline: Draws a line around elements, outside the border (
outline
). - Properties:
outline-width
: Thickness of the outline.outline-style
: Style of the outline (e.g.,solid
,dashed
).outline-color
: Color of the outline.outline-offset
: Space between the outline and the border.
Syntax:
div {
outline-width: 3px;
outline-style: dotted;
outline-color: blue;
outline-offset: 5px;
}
Code Example:
input {
outline: 2px dashed green;
}
Exercise:
- Add an outline to an
<input>
element with different styles, colors, and widths.
CSS Selectors
- Selectors: Target specific HTML elements to apply styles.
- Element Selector: Selects elements by tag name (e.g.,
p
). - Class Selector: Selects elements with a specific class
(
.class-name
). - ID Selector: Selects elements with a specific ID (
#id-name
). - Attribute Selector: Selects elements based on attributes
(
[type="text"]
).
- Element Selector: Selects elements by tag name (e.g.,
Syntax:
/* Element Selector */
p {
color: blue;
}
/* Class Selector */
.button {
background-color: yellow;
}
/* ID Selector */
#header {
font-size: 24px;
}
/* Attribute Selector */
input[type="text"] {
border: 1px solid gray;
}
Code Example:
h2 {
color: purple;
}
.highlight {
background-color: yellow;
}
#unique {
margin: 10px;
}
Exercise:
- Apply different styles using element, class, ID, and attribute selectors. For example, create a class for buttons, an ID for a header, and style text inputs with an attribute selector.
CSS Alignment (Text/Image/Content)
- Text Alignment: Align text within its container (
text-align
). - Vertical Alignment: Align inline or table-cell elements
(
vertical-align
). - Image Alignment: Align images within text or containers (
float
,display
).
Syntax:
/* Text Alignment */
p {
text-align: center; /* Options: left, right, center, justify */
}
/* Vertical Alignment */
img {
vertical-align: middle; /* Options: baseline, sub, super, top, middle, bottom */
}
/* Image Alignment */
img {
float: left; /* Options: left, right, none */
}
Code Example:
h1 {
text-align: right;
}
img {
vertical-align: top;
float: right;
}
Exercise:
- Align text to the center, an image to the right, and adjust vertical alignment for inline elements.
CSS Width & Height
- Width and Height: Set dimensions of elements (
width
,height
). - Units: Use pixels (
px
), percentages (%
), viewport units (vw
,vh
).
Syntax:
div {
width: 50%; /* Percentage */
height: 200px; /* Pixels */
}
Code Example:
.container {
width: 100vw; /* Full viewport width */
height: 100vh; /* Full viewport height */
}
Exercise:
- Define width and height for a
<div>
using different units, such as pixels and percentages.
CSS Text & Fonts
- Text Properties: Style text (
font-family
,font-size
,font-weight
,line-height
,text-transform
). - Font Families: Specify custom and web-safe fonts.
Syntax:
p {
font-family: Arial, sans-serif;
font-size: 16px;
font-weight: bold; /* Options: normal, bold, bolder, lighter, or numerical values */
line-height: 1.5;
text-transform: uppercase; /* Options: uppercase, lowercase, capitalize, none */
}
Code Example:
h2 {
font-family: 'Georgia', serif;
font-size: 24px;
text-transform: capitalize;
}
Exercise:
- Style text elements using different font families, sizes, weights, and transformations.
CSS Links
- Link States: Style links in different states (
:link
,:visited
,:hover
,:active
). - Pseudo-classes: Apply styles based on user interactions.
Syntax:
a:link {
color: blue; /* Unvisited link */
}
a:visited {
color: purple; /* Visited link */
}
a:hover {
color: red; /* Mouse over link */
}
a:active {
color: orange; /* Clicked link */
}
Code Example:
a {
text-decoration: none; /* Remove underline */
}
a:hover {
text-decoration: underline; /* Underline on hover */
}
Exercise:
- Style links to change colors based on their state, and remove or add underlines on hover.
CSS Buttons
- Button Styling: Customize buttons using various CSS properties
(
background-color
,border
,padding
,font-size
,cursor
). - Button States: Style different button states (
:hover
,:active
,:focus
).
Syntax:
button {
background-color: blue;
color: white;
border: none;
padding: 10px 20px;
font-size: 16px;
cursor: pointer; /* Change cursor on hover */
}
button:hover {
background-color: darkblue;
}
button:active {
background-color: navy;
}
Code Example:
.btn-primary {
background-color: green;
border-radius: 5px; /* Rounded corners */
}
.btn-primary:hover {
background-color: darkgreen;
}
Exercise:
- Create and style a button with different states (normal, hover, active). Experiment with background colors, padding, and border-radius.
CSS Flex Box
- Flexbox: Layout model for aligning items in containers
(
display: flex
). - Properties:
flex-direction
: Defines direction of flex items (e.g.,row
,column
).justify-content
: Aligns items horizontally (flex-start
,center
,space-between
,space-around
).align-items
: Aligns items vertically (flex-start
,center
,baseline
,stretch
).
Syntax:
.container {
display: flex;
flex-direction: row; /* or column */
justify-content: center;
align-items: center;
}
Code Example:
.flex-container {
display: flex;
flex-direction: column;
justify-content: space-between;
align-items: center;
}
.flex-item {
margin: 10px;
}
Exercise:
- Create a flex container and align items using different flexbox properties.
CSS Forms
- Form Styling: Customize form elements (
input
,textarea
,select
,button
). - Properties: Use properties like
padding
,border
,background
,font-size
to style form controls.
Syntax:
input, textarea, select, button {
padding: 10px;
border: 1px solid #ccc;
font-size: 16px;
}
Code Example:
input[type="text"] {
border-radius: 5px; /* Rounded corners */
}
textarea {
resize: vertical; /* Allow vertical resizing only */
}
button {
background-color: #4CAF50; /* Green */
color: white;
}
Exercise:
- Style various form elements (text fields, text areas, buttons) with padding, border, and background color.
CSS Lists
- List Styling: Customize list appearance (
list-style-type
,list-style-position
,list-style-image
). - Properties:
list-style-type
: Defines bullet or number style (e.g.,disc
,circle
,square
,decimal
).list-style-position
: Position of list marker (inside
,outside
).list-style-image
: Custom image for list marker.
Syntax:
ul {
list-style-type: circle;
list-style-position: inside;
}
ol {
list-style-type: decimal;
}
ul.custom {
list-style-image: url('bullet.png');
}
Code Example:
ul {
list-style-type: square;
padding-left: 20px;
}
ol {
list-style-type: lower-alpha;
}
Exercise:
- Style an unordered list and an ordered list with different list styles, positions, and custom images.
CSS Media Queries
- Media Queries: Apply styles based on device characteristics (e.g., screen width).
- Usage: Create responsive designs by targeting specific devices or screen sizes.
Syntax:
/* Styles for devices with a max-width of 600px */
@media (max-width: 600px) {
body {
background-color: lightyellow;
}
.container {
padding: 10px;
}
}
/* Styles for devices with a min-width of 601px */
@media (min-width: 601px) {
body {
background-color: lightblue;
}
}
Code Example:
@media (max-width: 768px) {
.sidebar {
display: none; /* Hide sidebar on small screens */
}
}
@media (min-width: 769px) {
.sidebar {
display: block; /* Show sidebar on larger screens */
}
}
Exercise:
- Implement media queries to adjust styles for different screen sizes, such as hiding elements on smaller screens or changing layouts.