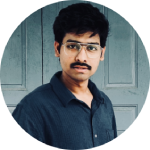
HTML Beginner Course
- HTML Introduction
- HTML Structure
- HTML Headings
- HTML Paragraphs
- HTML Text Formatting
- HTML Block and Inline Elements
- HTML Lists
- HTML Tables
- HTML Links
- HTML Attributes
- HTML Images
- HTML Self-Closing Elements
- HTML Video
- HTML Audio
- HTML Buttons
- HTML Forms: Text Fields
- HTML Forms: Text Area
- HTML Forms: Radio Buttons
- HTML Forms: Check Boxes
- HTML Forms: Drop Downs
- HTML Favicon
- HTML Symbols
- HTML Emojis
- HTML Semantics
- HTML iFrame/Youtube
Introduction to HTML
- HTML (HyperText Markup Language): The standard language for creating web pages.
- Basic structure: HTML documents consist of elements represented by tags.
- Tags: Surround content and apply meaning (e.g.,
<p>
,<h1>
,<div>
).
Syntax:
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
</head>
<body>
<p>This is a paragraph.</p>
</body>
</html>
Exercise:
- Create a basic HTML page with a title and a paragraph.
HTML Structure
- DOCTYPE declaration: Tells the browser the version of HTML (e.g.,
<!DOCTYPE html>
). - HTML document structure:
<html>
: Root element.<head>
: Contains meta-information (e.g., title, links to CSS).<body>
: Contains the content of the page.
Syntax:
<!DOCTYPE html>
<html>
<head>
<title>My Webpage</title>
</head>
<body>
<h1>Welcome to My Webpage</h1>
<p>This is an example of HTML structure.</p>
</body>
</html>
Exercise:
- Create an HTML document with a title and an
h1
heading inside the body.
HTML Headings
- Headings: Represent different levels of content (e.g.,
<h1>
to<h6>
). - Usage:
<h1>
is the highest level, typically used for main titles.
Syntax:
<h1>Main Heading</h1>
<h2>Subheading</h2>
<h3>Sub-subheading</h3>
Exercise:
- Create a webpage with all six levels of headings, displaying a content hierarchy.
HTML Paragraphs
- Paragraphs: Represent blocks of text (e.g.,
<p>
). - Usage: Used for regular text content in documents.
Syntax:
<p>This is a paragraph of text. HTML paragraphs are block-level elements.</p>
Exercise:
- Add multiple paragraphs to your webpage, explaining a topic of your choice.
HTML Text Formatting
- Text Formatting Elements: Used to style text (e.g.,
<strong>
,<em>
,<b>
,<i>
). - Purpose: Enhance text appearance and meaning.
Syntax:
<strong>Bold text</strong>
<em>Italic text</em>
<b>Bold text (less semantic)</b>
<i>Italic text (less semantic)</i>
Exercise:
- Format a block of text using different formatting elements and observe the changes.
HTML Block and Inline Elements
- Block Elements: Occupy the full width of their parent container (e.g.,
<div>
,<p>
,<h1>
). - Examples:
<div>
,<h1>
,<p>
,<ul>
,<table>
.
Syntax:
<div>
<h1>Block Level Element</h1>
<p>This is a paragraph inside a div.</p>
</div>
Exercise:
- Create a webpage with a
div
containing a heading and a paragraph.
HTML Lists
- Unordered Lists: Create lists with bullets (
<ul>
). - Ordered Lists: Create lists with numbers (
<ol>
). - List Items: Represent individual list items (
<li>
).
Syntax:
<ul>
<li>Item 1</li>
<li>Item 2</li>
</ul>
<ol>
<li>First item</li>
<li>Second item</li>
</ol>
Exercise:
- Create both an unordered and an ordered list on your webpage with at least three items each.
HTML Tables
- Tables: Display tabular data (
<table>
). - Table Rows: Represent each row (
<tr>
). - Table Headers: Define headers (
<th>
). - Table Data: Represent data cells (
<td>
).
Syntax:
<table>
<tr>
<th>Header 1</th>
<th>Header 2</th>
</tr>
<tr>
<td>Data 1</td>
<td>Data 2</td>
</tr>
</table>
Exercise:
- Create a table with at least two rows and two columns, including headers.
HTML Links
- Links: Create hyperlinks to navigate between pages (
<a>
). - Attributes:
href
specifies the URL,target
controls how the link opens (e.g.,_blank
).
Syntax:
<a href="https://www.example.com" target="_blank">Visit Example</a>
Exercise:
- Add a link to your webpage that points to an external website and opens in a new tab.
HTML Attributes
- Attributes: Provide additional information about HTML elements (e.g.,
id
,class
,src
,alt
). - Usage: Modify the behavior and appearance of elements.
Syntax:
<img src="image.jpg" alt="Description of image">
<a href="https://www.example.com" class="link">Example Link</a>
Exercise:
- Add an image with
src
andalt
attributes and a link with aclass
attribute to your webpage.
HTML Images
- Images: Display visual content (
<img>
). - Attributes:
src
specifies the image URL,alt
provides alternative text,width
andheight
set dimensions.
Syntax:
<img src="image.jpg" alt="Description of the image" width="300" height="200">
Exercise:
- Insert an image into your webpage with a descriptive
alt
attribute and adjust its size usingwidth
andheight
.
HTML Self-Closing Elements
- Self-Closing Tags: HTML5 allows certain elements to be self-closing (e.g.,
<img>
,<br>
,<hr>
). - Usage: These tags do not require a closing counterpart.
Syntax:
<img src="logo.png" alt="Logo">
<br>
<hr>
Exercise:
- Add a horizontal rule and a line break to your webpage, and include an image using a self-closing tag.
HTML Video
- Video: Embed video content (
<video>
). - Attributes:
src
specifies the video file,controls
adds playback controls,autoplay
starts video automatically.
Syntax:
<video src="video.mp4" controls width="640" height="360">
Your browser does not support the video tag.
</video>
Exercise:
- Embed a video into your webpage and add playback controls. Ensure you provide a fallback message for unsupported browsers.
HTML Audio
- Audio: Embed audio content (
<audio>
). - Attributes:
src
specifies the audio file,controls
adds playback controls,autoplay
starts audio automatically.
Syntax:
<audio src="audio.mp3" controls>
Your browser does not support the audio tag.
</audio>
Exercise:
- Add an audio file to your webpage with playback controls and a fallback message for unsupported browsers.
HTML Buttons
- Buttons: Create interactive buttons (
<button>
). - Types:
type
attribute specifies button behavior (submit
,reset
,button
).
Syntax:
<button type="button">Click Me</button>
<button type="submit">Submit</button>
<button type="reset">Reset</button>
Exercise:
- Create three buttons on your webpage with different types (
button
,submit
,reset
) and add simple JavaScript alerts to demonstrate their functionality.
HTML Forms: Text Fields
- Text Fields: Allow users to enter text
(
<input type="text">
). - Attributes:
name
assigns a name to the input field,placeholder
provides a hint.
Syntax:
<form>
<label for="username">Username:</label>
<input type="text" id="username" name="username" placeholder="Enter your username">
</form>
Exercise:
- Create a form with a text field for entering a username, and use a placeholder to guide the user.
HTML Forms: Text Area
- Text Area: Allows multi-line text input (
<textarea>
). - Attributes:
rows
andcols
define the size,name
assigns a name.
Syntax:
<form>
<label for="message">Message:</label>
<textarea id="message" name="message" rows="4" cols="50" placeholder="Enter your message"></textarea>
</form>
Exercise:
- Add a text area to your form where users can enter a message, specifying the number of rows and columns.
HTML Forms: Radio Buttons
- Radio Buttons: Allow selection of one option from a set
(
<input type="radio">
). - Attributes:
name
groups radio buttons,value
specifies the option value.
Syntax:
<form>
<label><input type="radio" name="gender" value="male"> Male</label>
<label><input type="radio" name="gender" value="female"> Female</label>
</form>
Exercise:
- Create a form with radio buttons for selecting a gender. Ensure all buttons share the same
name
attribute.
HTML Forms: Check Boxes
- Check Boxes: Allow multiple selections from a set
(
<input type="checkbox">
). - Attributes:
name
groups checkboxes,value
specifies the option value.
Syntax:
<form>
<label><input type="checkbox" name="hobby" value="reading"> Reading</label>
<label><input type="checkbox" name="hobby" value="traveling"> Traveling</label>
</form>
Exercise:
- Create a form with checkboxes for selecting hobbies. Ensure each checkbox has a unique value.
HTML Forms: Drop Downs
- Drop Down Menus: Allow selection from a list (
<select>
with<option>
). - Attributes:
name
assigns a name,value
specifies the option value.
Syntax:
<form>
<label for="car">Choose a car:</label>
<select id="car" name="car">
<option value="volvo">Volvo</option>
<option value="saab">Saab</option>
<option value="fiat">Fiat</option>
<option value="audi">Audi</option>
</select>
</form>
Exercise:
- Add a drop-down menu to your form that allows users to select a car model from a list of options.
HTML Favicon
- Favicon: Small icon displayed in the browser tab
(
<link rel="icon">
). - Attributes:
href
specifies the icon file location,type
specifies the file type.
Syntax:
<head>
<link rel="icon" href="favicon.ico" type="image/x-icon">
</head>
Exercise:
- Add a favicon to your webpage by linking to an icon file.
HTML Symbols
- Symbols: Special characters represented by HTML entities (e.g.,
©
,&
). - Usage: Display characters that are otherwise reserved or special.
Syntax:
<p>© 2024 Your Company</p>
<p>& More Symbols</p>
Exercise:
- Add a paragraph to your webpage using HTML entities for copyright and ampersand symbols.
HTML Emojis
- Emojis: Use Unicode characters to add emojis to your text.
- Usage: Enhance visual appeal and expressiveness.
Syntax:
<p>Have a great day! 😊</p>
Exercise:
- Include several emojis in a paragraph to convey a message or emotion.
HTML Semantics
- Semantics: Use HTML elements that clearly describe their meaning (e.g.,
<header>
,<footer>
,<article>
,<section>
). - Purpose: Improve readability and accessibility.
Syntax:
<header>
<h1>Page Title</h1>
</header>
<main>
<section>
<h2>Section Title</h2>
<p>Section content goes here.</p>
</section>
</main>
<footer>
<p>Footer content.</p>
</footer>
Exercise:
- Structure a webpage using semantic elements to define the header, main content, and footer.
HTML iFrame/Youtube
- iFrame: Embed external content within a page (
<iframe>
). - Attributes:
src
specifies the URL of the content,width
andheight
set dimensions.
Syntax:
<iframe src="https://www.youtube.com/embed/dQw4w9WgXcQ" width="560" height="315" frameborder="0" allowfullscreen></iframe>
Exercise:
- Embed a YouTube video on your webpage using an iframe. Adjust the width and height as needed.