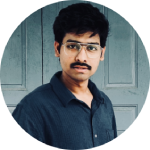
JavaScript Programming Beginner Course
- Basics of Programming using JavaScript
- JavaScript Introduction
- JavaScript in HTML Page
- JavaScript Variables
- JavaScript Data Types
- JavaScript Arithmetic Operators
- JavaScript Assignment Operators
- JavaScript Functions
- JavaScript Conditional Statements
- JavaScript Loops
- JavaScript Comments
- JavaScript Objects
- JavaScript Strings
- JavaScript Arrays
- JavaScript JSON
- JavaScript Debugging
- JavaScript integration with HTML Pages
- HTML DOM Introduction
- JavaScript Reading the HTML
- JavaScript Updating the HTML
- JavaScript Reading the CSS
- JavaScript Updating the CSS
- JavaScript Events and EventListener
Basics of Programming using JavaScript
Overview:
-
What is Programming?
- Writing instructions for a computer to perform specific tasks.
- Programming involves understanding algorithms and logic.
-
Introduction to JavaScript:
- JavaScript is a versatile, high-level programming language used for web development.
- It allows developers to create dynamic and interactive web content.
Key Concepts:
- Variables: Storage for data values.
- Data Types: Different types of data such as numbers, strings, etc.
- Operators: Symbols that perform operations on variables and values.
- Functions: Blocks of code designed to perform a particular task.
- Conditional Statements: Code structures that execute based on certain conditions.
- Loops: Code structures that repeat a block of code multiple times.
Examples:
// Variable declaration and assignment
let message = "Hello, World!";
// Function declaration
function greet(name) {
return "Hello, " + name + "!";
}
// Calling the function
console.log(greet("Alice")); // Outputs: Hello, Alice!
// Conditional statement
if (message === "Hello, World!") {
console.log("The message is correct.");
}
// Loop
for (let i = 0; i < 5; i++) {
console.log(i); // Outputs numbers 0 through 4
}
Exercises:
- Write a function that returns the sum of two numbers.
- Create a loop that prints numbers 1 through 10.
- Write a conditional statement that checks if a variable holds a value greater than 10 and logs a message if true.
Real-World Scenario:
- Creating a Simple Calculator: Use JavaScript to build a calculator that performs basic arithmetic operations like addition, subtraction, multiplication, and division.
JavaScript Introduction
Overview:
-
History and Evolution:
- Developed by Brendan Eich in 1995.
- Initially called Mocha, then LiveScript, and finally JavaScript.
-
JavaScript's Role in Web Development:
- Enhances HTML and CSS by adding interactivity and dynamic content.
Key Concepts:
- ECMAScript Standard: The specification JavaScript follows.
- Browser Compatibility: Different browsers may have slightly different implementations.
Examples:
<!DOCTYPE html>
<html>
<head>
<title>JavaScript Introduction</title>
</head>
<body>
<h1>Welcome to JavaScript</h1>
<script>
// JavaScript code
console.log("Hello, JavaScript!");
</script>
</body>
</html>
Exercises:
- Create an HTML file with an embedded JavaScript code that displays "Welcome to JavaScript" in the console.
- Modify the script to show an alert box with the message "Hello World!".
Real-World Scenario:
- Creating Interactive Elements: Use JavaScript to make a button that shows an alert when clicked.
JavaScript in HTML Page
Overview:
- Incorporating JavaScript:
- Inline, Internal, and External JavaScript.
- Inline JavaScript: Directly within HTML elements.
- Internal JavaScript: Inside
<script>
tags within an HTML file. - External JavaScript: Linked via an external
.js
file.
Examples:
<!DOCTYPE html>
<html>
<head>
<title>JavaScript in HTML</title>
</head>
<body>
<h1>JavaScript in HTML</h1>
<!-- Internal JavaScript -->
<script>
document.write("Hello from internal JavaScript!");
</script>
<!-- External JavaScript -->
<script src="script.js"></script>
</body>
</html>
script.js
console.log("Hello from external JavaScript!");
Exercises:
- Create an HTML file with internal JavaScript that changes the background color of the page.
- Link an external JavaScript file that displays the current date and time in the console.
Real-World Scenario:
- Interactive Web Page: Use JavaScript to validate form input and provide instant feedback to the user.
JavaScript Variables
Overview:
- Variable Declaration:
var
: Function-scoped variable.let
: Block-scoped variable.const
: Block-scoped, read-only variable.
Examples:
// Variable declarations
var oldVariable = "Old"; // Function scope
let newVariable = "New"; // Block scope
const constantVariable = "Constant"; // Block scope and read-only
// Using variables
console.log(oldVariable);
console.log(newVariable);
console.log(constantVariable);
Exercises:
- Declare three variables: one using
var
, one usinglet
, and one usingconst
. Print their values. - Attempt to reassign a value to a
const
variable and observe the result.
Real-World Scenario:
- Dynamic Content Update: Use variables to store user input and update the content of a web page based on that input.
JavaScript Data Types
Overview:
-
Primitive Data Types:
- String: Represents text. Example:
"Hello"
- Number: Represents numerical values. Example:
42
- Boolean: Represents true or false. Example:
true
- Undefined: Represents a variable that has not been assigned a value.
- Null: Represents the intentional absence of any value.
- Symbol: Represents a unique identifier.
- BigInt: Represents large integers.
- String: Represents text. Example:
-
Non-Primitive Data Types:
- Object: Represents a collection of key-value pairs.
- Array: Represents a list-like collection of values.
Examples:
// Primitive data types
let name = "Alice"; // String
let age = 30; // Number
let isStudent = true; // Boolean
let address; // Undefined
let score = null; // Null
// Non-primitive data types
let person = { name: "Alice", age: 30 }; // Object
let numbers = [1, 2, 3, 4, 5]; // Array
console.log(name, age, isStudent, address, score);
console.log(person);
console.log(numbers);
Exercises:
- Create variables of each data type and print their types using
typeof
. - Build an object that represents a book with properties like title, author, and year.
Real-World Scenario:
- User Profile: Store user information such as name, age, and preferences in an object, and update or display this information dynamically on a web page.
JavaScript Arithmetic Operators
Overview:
- Purpose: Perform arithmetic operations on numerical values.
Operators:
- Addition (
+
): Adds two numbers. - Subtraction (
-
): Subtracts one number from another. - Multiplication (
*
): Multiplies two numbers. - Division (
/
): Divides one number by another. - Modulus (
%
): Returns the remainder of a division. - Exponentiation (
**
): Raises a number to the power of another.
Examples:
let a = 10;
let b = 5;
console.log(a + b); // Addition: 15
console.log(a - b); // Subtraction: 5
console.log(a * b); // Multiplication: 50
console.log(a / b); // Division: 2
console.log(a % b); // Modulus: 0
console.log(a ** b); // Exponentiation: 100000
Exercises:
- Create a script that calculates the area of a rectangle (length * width) and logs it to the console.
- Write a function that takes two numbers and returns their average using arithmetic operations.
Real-World Scenario:
- Expense Calculator: Create a calculator that computes the total cost of items purchased, including taxes and discounts.
JavaScript Assignment Operators
Overview:
- Purpose: Assign values to variables and perform operations in a concise manner.
Operators:
- Assignment (
=
): Assigns a value to a variable. - Addition Assignment (
+=
): Adds a value to a variable and assigns the result. - Subtraction Assignment (
-=
): Subtracts a value from a variable and assigns the result. - Multiplication Assignment (
*=
): Multiplies a variable by a value and assigns the result. - Division Assignment (
/=
): Divides a variable by a value and assigns the result. - Modulus Assignment (
%=
): Applies modulus operation and assigns the result.
Examples:
let x = 10;
x += 5; // Equivalent to x = x + 5, x is now 15
x -= 3; // Equivalent to x = x - 3, x is now 12
x *= 2; // Equivalent to x = x * 2, x is now 24
x /= 4; // Equivalent to x = x / 4, x is now 6
x %= 5; // Equivalent to x = x % 5, x is now 1
Exercises:
- Write a script that uses assignment operators to update the value of a variable representing a bank account balance.
- Create a function that uses different assignment operators to calculate the final price of a product after applying discounts and taxes.
Real-World Scenario:
- Budget Tracker: Build a simple budget tracker that updates and displays remaining balance after adding expenses or income.
JavaScript Functions
Overview:
- Purpose: Encapsulate reusable blocks of code that perform specific tasks.
Function Declaration:
- Syntax:
function functionName(parameters) {
// Code to execute
}
- Example:
function add(a, b) {
return a + b;
}
console.log(add(5, 3)); // Outputs: 8
Function Expressions:
- Syntax:
const multiply = function(x, y) {
return x * y;
};
console.log(multiply(4, 6)); // Outputs: 24
Arrow Functions:
- Syntax:
const divide = (a, b) => a / b;
console.log(divide(12, 4)); // Outputs: 3
Exercises:
- Create a function that takes a name as a parameter and returns a greeting message.
- Write a function that calculates and returns the factorial of a number.
Real-World Scenario:
- Form Validation: Use functions to validate user input in a form, such as checking if an email address is correctly formatted.
JavaScript Conditional Statements
Overview:
- Purpose: Execute different code based on conditions.
Types of Conditional Statements:
-
if
Statement:- Syntax:
if (condition) { // Code to execute if condition is true }
-
if...else
Statement:- Syntax:
if (condition) { // Code to execute if condition is true } else { // Code to execute if condition is false }
-
else if
Statement:- Syntax:
if (condition1) { // Code to execute if condition1 is true } else if (condition2) { // Code to execute if condition2 is true } else { // Code to execute if none of the conditions are true }
-
switch
Statement:- Syntax:
switch (expression) { case value1: // Code to execute if expression matches value1 break; case value2: // Code to execute if expression matches value2 break; default: // Code to execute if no case matches }
Examples:
let day = 2;
if (day === 1) {
console.log("Monday");
} else if (day === 2) {
console.log("Tuesday");
} else {
console.log("Other day");
}
// Switch case example
let fruit = "apple";
switch (fruit) {
case "apple":
console.log("This is an apple.");
break;
case "banana":
console.log("This is a banana.");
break;
default:
console.log("Unknown fruit.");
}
Exercises:
- Write a script that uses
if...else
statements to check if a number is positive, negative, or zero. - Create a function that uses a
switch
statement to return the name of the month based on a number (1-12).
Real-World Scenario:
- User Role Access: Use conditional statements to manage access to different parts of a web application based on user roles (e.g., admin, editor, viewer).
JavaScript Loops
Overview:
- Purpose: Repeat a block of code multiple times.
Types of Loops:
-
for
Loop:- Syntax:
for (initialization; condition; increment/decrement) { // Code to execute }
-
while
Loop:- Syntax:
while (condition) { // Code to execute }
-
do...while
Loop:- Syntax:
do { // Code to execute } while (condition);
Examples:
// For loop
for (let i = 0; i < 5; i++) {
console.log(i); // Outputs numbers 0 through 4
}
// While loop
let j = 0;
while (j < 5) {
console.log(j); // Outputs numbers 0 through 4
j++;
}
// Do...while loop
let k = 0;
do {
console.log(k); // Outputs numbers 0 through 4
k++;
} while (k < 5);
Exercises:
- Write a
for
loop that prints the first 10 even numbers. - Create a
while
loop that calculates the sum of numbers from 1 to 100.
Real-World Scenario:
- Data Processing: Use loops to iterate over and process items in a shopping cart, such as calculating the total price of all items.
JavaScript Comments
Overview:
- Purpose: Provide explanations within the code to make it easier to understand and maintain.
Types of Comments:
- Single-Line Comments: Use
//
for comments that occupy a single line. - Multi-Line Comments: Use
/* ... */
for comments that span multiple lines.
Examples:
// This is a single-line comment
/*
This is a multi-line comment
It can span multiple lines
*/
let x = 10; // Initialize x with 10
Exercises:
- Add comments to a piece of code to explain what each section does.
- Write a JavaScript function with comments explaining its purpose, parameters, and return value.
Real-World Scenario:
- Code Documentation: Use comments to document complex logic in your code, making it easier for other developers (or yourself) to understand and maintain it.
JavaScript Objects
Overview:
- Purpose: Store collections of key-value pairs where keys are strings and values can be any data type.
Key Concepts:
-
Creating Objects:
- Literal Notation:
let person = { name: "Alice", age: 30, greet: function() { return "Hello, " + this.name; } };
-
Accessing Object Properties:
console.log(person.name); // Outputs: Alice console.log(person["age"]); // Outputs: 30
-
Adding/Updating Properties:
person.email = "alice@example.com"; person.age = 31;
-
Deleting Properties:
delete person.email;
Examples:
let car = {
make: "Toyota",
model: "Corolla",
year: 2020,
start: function() {
return "Car started";
}
};
console.log(car.make); // Outputs: Toyota
console.log(car.start()); // Outputs: Car started
Exercises:
- Create an object that represents a book with properties for title, author, and publication year. Add a method to display the book's details.
- Write a function that takes an object representing a student and returns a formatted string with their name and grade.
Real-World Scenario:
- User Profile Management: Use objects to store and manage user profile information in a web application, including their name, email, and preferences.
JavaScript Strings
Overview:
- Purpose: Represent and manipulate text data.
Key Concepts:
-
String Creation:
let str1 = "Hello, World!"; let str2 = 'JavaScript is fun';
-
String Concatenation:
let greeting = "Hello"; let name = "Alice"; let message = greeting + ", " + name + "!";
-
String Methods:
- Length:
str.length
- Uppercase/Lowercase:
str.toUpperCase()
,str.toLowerCase()
- Trim:
str.trim()
- Substring:
str.substring(start, end)
- Replace:
str.replace(search, replace)
- Length:
Examples:
let message = " JavaScript is awesome! ";
console.log(message.trim()); // Outputs: JavaScript is awesome!
console.log(message.toUpperCase()); // Outputs: JAVASCRIPT IS AWESOME!
console.log(message.substring(0, 10)); // Outputs: JavaScript
console.log(message.replace("awesome", "fantastic")); // Outputs: JavaScript is fantastic!
Exercises:
- Write a script that takes a string and logs its length, uppercase version, and the first 5 characters.
- Create a function that replaces all occurrences of a word in a string with another word.
Real-World Scenario:
- Text Formatting: Use string methods to format user input for consistent display, such as capitalizing names or formatting addresses.
JavaScript Arrays
Overview:
- Purpose: Store and manipulate collections of items, such as lists of values.
Key Concepts:
-
Creating Arrays:
let numbers = [1, 2, 3, 4, 5]; let names = ["Alice", "Bob", "Charlie"];
-
Accessing Elements:
console.log(numbers[0]); // Outputs: 1 console.log(names[1]); // Outputs: Bob
-
Array Methods:
- Push:
arr.push(item)
– Adds an item to the end. - Pop:
arr.pop()
– Removes the last item. - Shift:
arr.shift()
– Removes the first item. - Unshift:
arr.unshift(item)
– Adds an item to the beginning. - Slice:
arr.slice(start, end)
– Returns a new array with a portion of the original array. - Splice:
arr.splice(start, deleteCount, item1, item2, ...)
– Adds/removes items at a specific index.
- Push:
Examples:
let fruits = ["apple", "banana", "cherry"];
fruits.push("date");
console.log(fruits); // Outputs: ["apple", "banana", "cherry", "date"]
fruits.pop();
console.log(fruits); // Outputs: ["apple", "banana", "cherry"]
let slicedFruits = fruits.slice(1, 3);
console.log(slicedFruits); // Outputs: ["banana", "cherry"]
Exercises:
- Write a script that creates an array of numbers, adds a new number, and removes the first number.
- Create a function that takes an array of strings and returns a new array with all strings converted to uppercase.
Real-World Scenario:
- Todo List: Use arrays to manage a list of tasks, allowing users to add, remove, and view their tasks.
JavaScript JSON
Overview:
- Purpose: Exchange data between a server and a web application in a structured format.
Key Concepts:
-
JSON Format:
- JSON (JavaScript Object Notation) is a lightweight data-interchange format that is easy for humans to read and write and easy for machines to parse and generate.
- Example:
{ "name": "Alice", "age": 30, "email": "alice@example.com" }
-
Parsing JSON:
let jsonString = '{"name": "Alice", "age": 30}'; let obj = JSON.parse(jsonString); console.log(obj.name); // Outputs: Alice
-
Stringifying JSON:
let user = { name: "Alice", age: 30 }; let jsonString = JSON.stringify(user); console.log(jsonString); // Outputs: {"name":"Alice","age":30}
Examples:
let jsonData = '{"name": "Bob", "age": 25}';
let dataObject = JSON.parse(jsonData);
console.log(dataObject.name); // Outputs: Bob
let newUser = { name: "Charlie", age: 35 };
let jsonOutput = JSON.stringify(newUser);
console.log(jsonOutput); // Outputs: {"name":"Charlie","age":35}
Exercises:
- Parse a JSON string representing a list of products and log each product's name.
- Convert a JavaScript object with user details into a JSON string and log it.
Real-World Scenario:
- API Data Handling: Use JSON to fetch and process data from a web API, such as retrieving user information or updating settings.
JavaScript Debugging
Overview:
- Purpose: Identify and fix errors or issues in JavaScript code to ensure it runs correctly.
Key Techniques:
-
Using
console.log
: Print messages to the console to check variable values and program flow.let x = 10; console.log(x); // Outputs: 10
-
Breakpoints: Set breakpoints in browser developer tools to pause execution and inspect code.
-
Debugger Statement:
function test() { debugger; // Code execution will pause here console.log("Testing..."); } test();
-
Error Handling: Use
try...catch
blocks to manage runtime errors.try { let result = riskyFunction(); } catch (error) { console.error("An error occurred:", error); }
Examples:
function divide(a, b) {
if (b === 0) {
console.error("Cannot divide by zero.");
return;
}
return a / b;
}
console.log(divide(10, 2)); // Outputs: 5
console.log(divide(10, 0)); // Outputs: Cannot divide by zero.
JavaScript Integration with HTML Pages
Overview:
- Purpose: Embed JavaScript code within HTML to enhance web page functionality.
Key Concepts:
-
Inline JavaScript:
- Directly within HTML elements using the
onclick
,onchange
, or other event attributes.
<button onclick="alert('Hello World!')">Click Me</button>
- Directly within HTML elements using the
-
Internal JavaScript:
- Within a
<script>
tag inside the HTML document.
<script> function greet() { alert("Hello from internal script!"); } </script> <button onclick="greet()">Click Me</button>
- Within a
-
External JavaScript:
- Linking to an external
.js
file using the<script>
tag with asrc
attribute.
<script src="script.js"></script>
- Linking to an external
Examples:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Integration</title>
<script src="app.js"></script>
</head>
<body>
<button onclick="showMessage()">Click Me</button>
</body>
</html>
// app.js
function showMessage() {
alert("Hello from external script!");
}
Exercises:
- Create an HTML page that uses both internal and external JavaScript to display messages and perform actions.
- Write a script that dynamically changes the content of a webpage based on user interaction.
Real-World Scenario:
- Interactive Forms: Use JavaScript to validate form inputs and provide immediate feedback to users.
HTML DOM Introduction
Overview:
- Purpose: Interact with and manipulate the structure and content of a web page using JavaScript.
Key Concepts:
- DOM (Document Object Model): Represents the page structure as a tree of nodes.
- Accessing Elements:
getElementById()
,getElementsByClassName()
,getElementsByTagName()
querySelector()
,querySelectorAll()
Examples:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>DOM Example</title>
</head>
<body>
<h1 id="header">Hello, World!</h1>
<script>
// Access element by ID
let header = document.getElementById('header');
header.textContent = "Hello, DOM!";
</script>
</body>
</html>
Exercises:
- Modify the content of an HTML element using JavaScript based on user interaction.
- Create a script that changes the style of an element when a button is clicked.
Real-World Scenario:
- Dynamic Content Updates: Use DOM manipulation to update content in real-time based on user actions, such as updating a profile or news feed.
JavaScript Reading the HTML
Overview:
- Purpose: Extract and use data from the HTML content using JavaScript.
Key Concepts:
- Reading Element Content:
textContent
,innerHTML
,innerText
- Reading Attributes:
getAttribute()
Examples:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Read HTML</title>
</head>
<body>
<p id="description" data-info="Additional Info">This is a paragraph.</p>
<script>
let paragraph = document.getElementById('description');
console.log(paragraph.textContent); // Outputs: This is a paragraph.
console.log(paragraph.getAttribute('data-info')); // Outputs: Additional Info
</script>
</body>
</html>
Exercises:
- Write a script to read and log the content and attributes of various HTML elements.
- Create a function that extracts and displays information from an HTML element based on user interaction.
Real-World Scenario:
- Content Extraction: Use JavaScript to read data from elements on a page and use it to populate or modify other parts of the web application.
JavaScript Updating the HTML
Overview:
- Purpose: Change the content and structure of HTML elements dynamically using JavaScript.
Key Concepts:
- Updating Content:
textContent
,innerHTML
- Updating Attributes:
setAttribute()
- Creating and Inserting Elements:
createElement()
,appendChild()
,insertBefore()
Examples:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Update HTML</title>
</head>
<body>
<div id="container"></div>
<script>
let container = document.getElementById('container');
container.textContent = "New content added dynamically.";
let newElement = document.createElement('p');
newElement.textContent = "This is a new paragraph.";
container.appendChild(newElement);
</script>
</body>
</html>
Exercises:
- Create a script that updates the content and attributes of HTML elements based on user input.
- Write a function that dynamically adds new elements to a webpage, such as a list of items.
Real-World Scenario:
- Dynamic User Interfaces: Use JavaScript to update user interfaces in response to user actions or external data, such as updating a chat interface or live data feed.
JavaScript Reading the CSS
Overview:
- Purpose: Access and read CSS properties applied to HTML elements.
Key Concepts:
- Reading Computed Styles:
getComputedStyle()
- Accessing Specific Properties:
style.propertyName
for inline styles
Examples:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Read CSS</title>
<style>
#box {
width: 100px;
height: 100px;
background-color: blue;
}
</style>
</head>
<body>
<div id="box"></div>
<script>
let box = document.getElementById('box');
let styles = getComputedStyle(box);
console.log(styles.width); // Outputs: 100px
console.log(styles.backgroundColor); // Outputs: rgb(0, 0, 255)
</script>
</body>
</html>
Exercises:
- Write a script to read and log the computed styles of various HTML elements.
- Create a function that checks and reports whether an element has a certain style applied.
Real-World Scenario:
- Style Analysis: Use JavaScript to analyze and report styles applied to elements, which can be useful for debugging or dynamic style adjustments.
JavaScript Updating the CSS
Overview:
- Purpose: Modify CSS properties of HTML elements dynamically using JavaScript.
Key Concepts:
- Inline Styles:
- Directly update the
style
property of an element.
- Directly update the
- Class Manipulation:
- Add or remove CSS classes using
classList
methods.
- Add or remove CSS classes using
Examples:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Update CSS</title>
<style>
#box {
width: 100px;
height: 100px;
background-color: red;
}
.blue-background {
background-color: blue;
}
</style>
</head>
<body>
<div id="box"></div>
<button onclick="changeStyle()">Change Style</button>
<script>
function changeStyle() {
let box = document.getElementById('box');
box.style.width = '200px';
box.style.height = '200px';
box.classList.add('blue-background');
}
</script>
</body>
</html>
Exercises:
- Create a script that updates the background color and dimensions of an HTML element based on user input.
- Write a function that toggles a CSS class on an element to apply different styles.
Real-World Scenario:
- Interactive Design: Use JavaScript to apply different styles in response to user interactions, such as changing themes or layouts.
JavaScript Events
Overview:
- Purpose: Handle and respond to user interactions or other events that occur in the browser.
Key Concepts:
-
Event Types:
- Mouse Events:
click
,mouseover
,mouseout
- Keyboard Events:
keydown
,keyup
- Form Events:
submit
,change
- Mouse Events:
-
Event Handling:
- Event Listeners:
addEventListener()
- Inline Event Handlers: Using attributes like
onclick
- Event Listeners:
Examples:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Events</title>
</head>
<body>
<button id="myButton">Click Me</button>
<script>
let button = document.getElementById('myButton');
button.addEventListener('click', function() {
alert('Button was clicked!');
});
</script>
</body>
</html>
Exercises:
- Write a script that handles a form submission event to prevent the default action and display a message instead.
- Create a function that responds to keyboard events by logging the key pressed.
Real-World Scenario:
- User Interaction: Use JavaScript to handle various user interactions on a webpage, such as form submissions, button clicks, or keyboard inputs.